As IPv4 is already exhausted, so many ISP is switching to a mixture of private IPv4 and public IPv6 network. So, it opened a scope for many to experiment with IPv6, share files or simply server a web page over IPv6.
But just for serving few static page over IPv6, most of you won't like to setup heavy weight servers like NGINX, Apache for that.
Previously I wrote about 5 simple web server, but most of them has no IPv6 support.
So, here's a simple python script as a basic IPv6 capable web server, based on python SimpleHTTPServer module.
Contents
The IPv6 web server script
Here's the script, written with python2.7.x series.
import socket from BaseHTTPServer import HTTPServer from SimpleHTTPServer import SimpleHTTPRequestHandler class MyHandler(SimpleHTTPRequestHandler): def do_GET(self): if self.path == '/ip': self.send_response(200) self.send_header('Content-type', 'text/html') self.end_headers() self.wfile.write('Your IP address is %s' % self.client_address[0]) return else: return SimpleHTTPRequestHandler.do_GET(self) class HTTPServerV6(HTTPServer): address_family = socket.AF_INET6 def main(): server = HTTPServerV6(('::', 8080), MyHandler) server.serve_forever() if __name__ == '__main__': main()
That's it, save the the script with any name, I saved it as simple_IPv6_web_server.py
.
You can change the port number to your desired port in the script.
Using Python 3
It's veven siompler when using python 3, using the http.server module.
python3 -m http.server --bind ::
In this context, :: denotes the IPv6 localhost. You can also define a specific IPv6 address and port, example below.
python3 -m http.server -b 2603:4000:3bd0::786:bbad:1e5d 8080
Here 2603:4000:3bd0::786:bbad:1e5d is an example IPv6 address and 8080 is the spesific port of your choice.
Running and testing the web server script
You can run the script with the python command,
python2.7 simple_IPv6_web_server.py
By default it binds all the the interfaces with IPv6 address to the port 8080, you can check with netstat
command or a web browser.

If there's a index.html file, it will be served as default page, unless there will be a directory listing of the pwd.
So, first find out your IPv6 address and construct the IPv6 URL like this, [your_IPv5_address]:8080
, a more prominent example could be like below.
http://[2405:205:6226:7b98:a067:64de:fc0b:f0]:8080/
.
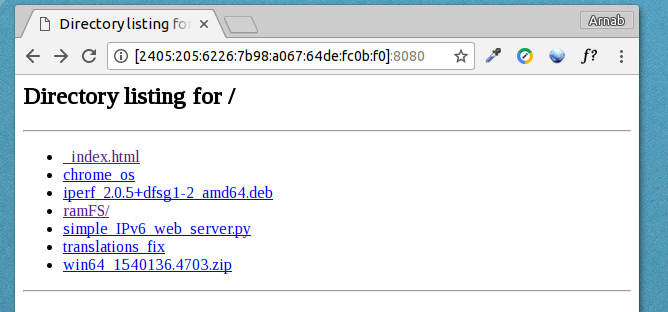
Credit and conclusion
A big thanks to the script author, Alexander Korobov, link to the original script here.
So, that's all about how you can create a simple IPv6 web server with python, I hope that's interesting enough.
If you have any question or suggestion, please leave comments.
Leave a Reply